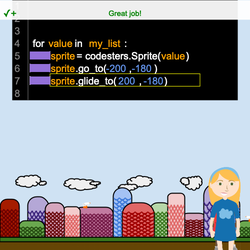
演示#3 :在这个例子中,您将能够运行整个循环来查看您的预测是否正确!
循环内的命令旁边有一个紫色块,如下所示: ····
To navigate the page using the TAB key, first press ESC to exit the code editor.
def hover():
x = stage.mouse_x()
y = stage.mouse_y()
if (x < -150 and x > -250) and (y < -200 and y > -250):
click_rect.set_opacity(.7)
rect_text.set_opacity(.7)
else:
click_rect.set_opacity(1)
rect_text.set_opacity(1)
stage.set_background("game")
code_back = codesters.Rectangle(0, 150, 450, 200, "black")
num_y = 240
for counter in range(8):
num = codesters.Text(str(counter +1), -215, num_y, "gray")
num_y -= 25
line = codesters.Line(-194, 250, -194, 50, "gray")
list_name = codesters.Text("my_list = [ , , ]", 13, 215, "white")
list_values = codesters.Text('"person1" "person2" "person11"', 55, 215, "lightgreen")
y = 165
x = 0
spacing = 25
line1 = codesters.Text("for in :", x - 90, y, "white")
line1a = codesters.Text("value my_list", x - 81, y, "orange")
indent = 40
y-= spacing
line2 = codesters.Text("= codesters.Sprite( )", x-20 + indent, y, "white")
line2a = codesters.Text('sprite value', x-50+ indent, y, "orange")
spacer1 = codesters.Rectangle(-170, y, indent, spacing, "mediumpurple")
y-=spacing
line3 = codesters.Text('.go_to( , )', x- 53+ indent, y, "white")
line3a = codesters.Text('sprite', x - 160+ indent, y, "orange")
line3b = codesters.Text('-200 -180', x -28+ indent ,y, "cornflowerblue")
spacer2 = codesters.Rectangle(-170, y, indent, spacing, "mediumpurple")
y-= spacing
line4 = codesters.Text('.glide_to( , )', x - 43+ indent, y, "white")
line4a = codesters.Text('sprite', x - 160+ indent, y, "orange")
line4b = codesters.Text('200 -180', x - 4+ indent, y, "cornflowerblue")
spacer3 = codesters.Rectangle(-170, y, indent, spacing, "mediumpurple")
guide_rect = codesters.Rectangle(0, -50, 500, 60, "lightblue")
guide = codesters.Text("The commands INSIDE a loop must be indented 4 spaces for the loop to run.", 0, -50)
click_rect = codesters.Rectangle(-200, -220, 125, 60, "green", "black")
rect_text = codesters.Text("Continue", -200, -220, "white")
stage.event_mouse_move(hover)
highlight = codesters.Rectangle(200, 215, 90, 25, "yellow")
highlight.set_opacity(.5)
highlight.hide()
highlight2 = codesters.Rectangle(0, 140, 300, 25, None, "yellow")
highlight2.hide()
spacers = [spacer1, spacer2, spacer3]
def click_a():
rect_text.set_text(' ')
click_rect.hide()
guide.set_text("First, let's see how to fix a broken loop. \nClick on the purple block to remove it.")
for spacer in spacers:
spacer.event_click(click_1)
def click_1():
global indent
for spacer in spacers:
spacer.hide()
rect_text.set_text(" ")
lines = [line2, line2a, line3, line3a, line3b, line4, line4a, line4b]
for i in lines:
i.set_x(i.get_x() - indent)
guide.set_text("Oh no! This code won't run! Click on the code to get to the beginning of the first command in the loop block.")
code_back.event_click(click_2)
def click_2():
guide.set_text("Notice the cursor before the code on line 5. Use the space bar to move the code 4 spaces to the right.")
cursor = codesters.Line(line2a.get_x() - 140, line2a.get_y() + 8, line2a.get_x() - 140, line2a.get_y() - 10, "white")
spaces = []
def space_bar():
new_x = cursor.get_x()
new_y = cursor.get_y()
if len(spaces) < 3:
red_space = codesters.Rectangle(new_x + 5, new_y, 10, 15, "red")
spaces.append(red_space)
cursor.set_x(red_space.get_right())
new_line2 = line2.get_x() + 9
new_line2a = line2a.get_x() + 9
line2.set_x(new_line2)
line2a.set_x(new_line2a)
guide.set_text("Press the space bar to move the line of code to the right!")
elif len(spaces) == 3:
new_x = cursor.get_x()
new_y = cursor.get_y()
purple_space = codesters.Rectangle(new_x + 5, new_y, 10, 15, "mediumpurple")
for i in spaces:
i.set_color("mediumpurple")
spaces.append(purple_space)
cursor.set_x(purple_space.get_right())
new_line2 = line2.get_x() + 9
new_line2a = line2a.get_x() + 9
line2.set_x(new_line2)
line2a.set_x(new_line2a)
guide.set_text("Great! Click on the next line of code to continue.")
code_back.event_click(click_3)
def space_bar_2():
guide.set_text("Try clicking on the next line above continue.")
stage.event_key("space", space_bar_2)
stage.event_key("space", space_bar)
def wrong_ans():
guide.set_text("Try clicking on the code above to continue!")
click_rect.event_click(wrong_ans)
def click_3():
guide.set_text("Notice the cursor before the code on line 6. Use the space bar to move the code 4 spaces to the right.")
cursor1 = codesters.Line(line3a.get_x() - 30, line3a.get_y() + 8, line3a.get_x() - 30, line3a.get_y() - 10, "white")
spaces = []
def space_bar():
new_x = cursor1.get_x()
new_y = cursor1.get_y()
if len(spaces) < 3:
red_space = codesters.Rectangle(new_x + 5, new_y, 10, 15, "red")
spaces.append(red_space)
cursor1.set_x(red_space.get_right())
new_line3 = line3.get_x() + 9
new_line3a = line3a.get_x() + 9
new_line3b = line3b.get_x() + 9
line3.set_x(new_line3)
line3a.set_x(new_line3a)
line3b.set_x(new_line3b)
guide.set_text("Press the space bar to move the line of code to the right!")
elif len(spaces) == 3:
new_x = cursor1.get_x()
new_y = cursor1.get_y()
purple_space = codesters.Rectangle(new_x + 5, new_y, 10, 15, "purple")
spaces.append(purple_space)
cursor1.set_x(purple_space.get_right())
new_line3 = line3.get_x() + 9
new_line3a = line3a.get_x() + 9
new_line3b = line3b.get_x() + 9
line3.set_x(new_line3)
line3a.set_x(new_line3a)
line3b.set_x(new_line3b)
for i in spaces:
i.set_color("mediumpurple")
guide.set_text("Great! Click on the next line of code to continue.")
code_back.event_click(click_4)
def space_bar_2():
guide.set_text("Try clicking on the next line above continue.")
stage.event_key("space", space_bar_2)
stage.event_key("space", space_bar)
def click_4():
guide.set_text("Notice the cursor before the code on line 6. Use the space bar to move the code 4 spaces to the right.")
cursor1 = codesters.Line(line4a.get_x() - 30, line4a.get_y() + 8, line4a.get_x() - 30, line4a.get_y() - 10, "white")
spaces = []
def space_bar():
new_x = cursor1.get_x()
new_y = cursor1.get_y()
if len(spaces) < 3:
red_space = codesters.Rectangle(new_x + 5, new_y, 10, 15, "red")
spaces.append(red_space)
cursor1.set_x(red_space.get_right())
new_line4 = line4.get_x() + 9
new_line4a = line4a.get_x() + 9
new_line4b = line4b.get_x() + 9
line4.set_x(new_line4)
line4a.set_x(new_line4a)
line4b.set_x(new_line4b)
guide.set_text("Press the space bar to move the line of code to the right!")
elif len(spaces) == 3:
new_x = cursor1.get_x()
new_y = cursor1.get_y()
purple_space = codesters.Rectangle(new_x + 5, new_y, 10, 15, "red")
spaces.append(purple_space)
cursor1.set_x(purple_space.get_right())
new_line4 = line4.get_x() + 9
new_line4a = line4a.get_x() + 9
new_line4b = line4b.get_x() + 9
line4.set_x(new_line4)
line4a.set_x(new_line4a)
line4b.set_x(new_line4b)
for i in spaces:
i.set_color("mediumpurple")
guide.set_text("Great! Click the rectangle to run the loop.")
rect_text.set_text("Continue")
click_rect.show()
click_rect.event_click(click_5)
code_back.event_click(delete_event)
def space_bar_2():
guide.set_text("Try clicking on the rectangle to run the loop.")
stage.event_key("space", space_bar_2)
stage.event_key("space", space_bar)
def click_5():
click_rect.hide()
rect_text.set_text(' ')
guide.set_text(" ")
stage.remove_sprite(guide_rect)
my_list = ["person1", "person2", "person11"]
highlight.show()
highlight2.show()
x_high = -45
highlight.set_x(x_high)
for i in my_list:
y_high2 = 140
highlight2.set_y(y_high2)
highlight.set_x(x_high)
sprite = codesters.Sprite(i)
stage.wait(.5)
y_high2 -= 25
highlight2.set_y(y_high2)
sprite.go_to(-200, -180)
stage.wait(.5)
y_high2 -= 25
highlight2.set_y(y_high2)
sprite.glide_to(200, -180)
stage.wait(.5)
x_high += 100
list_name.hide()
list_values.hide()
highlight.hide()
tester = TestManager()
tester.display_success_message("Great job!")
def delete_event():
pass
click_rect.event_click(click_a)
,